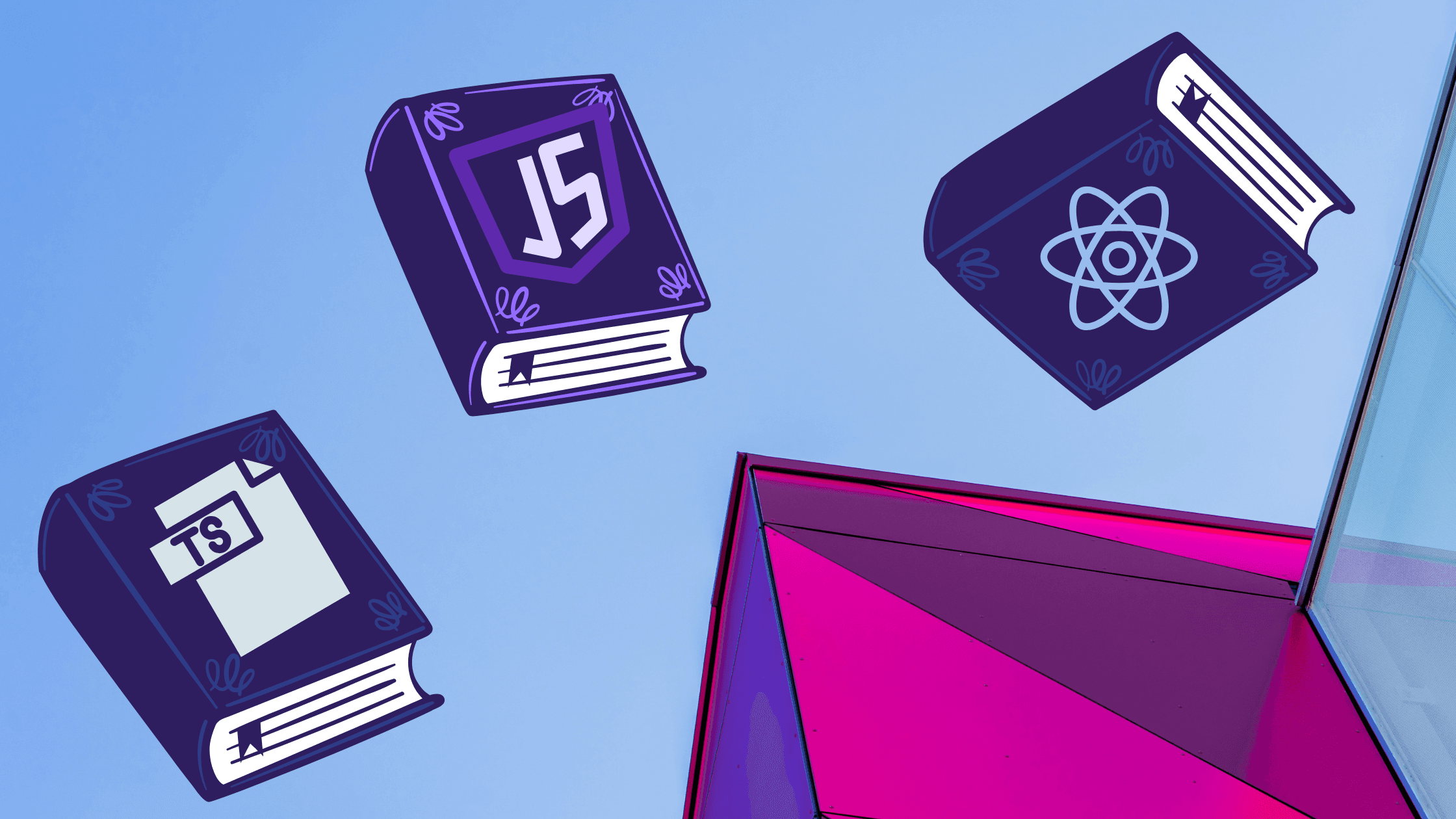
from November 2024 Study Group session
What is memoization?
the idea of caching the results of a function improve efficiency
usually use a dictionary as the caching object to store the results
in React, useMemo() and useCallback() can be used. The former is used to cache values; the latter is used for functions
useCallback() is for caching functions between renders. The main benefit being that child components that take those functions as props won’t need to re-render unnecessarily
Example:
function fibonacci(n, memo = {}) {
if (n <= 1) return n;
if (memo[n]) return memo[n];
memo[n] = fibonacci(n - 1, memo) + fibonacci(n - 2, memo);
return memo[n];
}
When to use useEffect vs useMemo?
The contents of a useEffect runs whenever the React component loads (if the dependencies array is []) OR when a state in the dependencies array is updated
useMemo is used when we want to cache the value returned from a function
What is local storage vs session storage and how do you access both?
localStorage doesn’t expire, sessionStorage clears when the page session ends - whenever a doc is loaded in a particular tab in the browser, a unique session gets created and assigned to that particular tab
What is an easy way to find the maximum or minimum number in an array in JavaScript?
Possible way is to sort and then take the 0 index for the min or last index for max
Math.min(...array) or Math.max(...array)
Optimized? way - do not sort or use built in methods - just for loop through the array and keep track of a max or min (usually sorting adds time complexity)
What is the best way to check that a value IS a number in JavaScript?
typeof VALUE === number
Number.isInteger(value)
What are some ways I can speed up the performance of my web app, from a front-end perspective? (“A page is loading slowly. What do I check?
Fewer images/videos
Smaller images
Make sure your javascript is performant
Only load stylesheets, JS files, etc that must be loaded for the page
Reduce complex CSS selectors
Lazy loading images
Lower resolution images/videos
Fewer third-party libraries
Reduce HTTP requests
Let’s say some users have low-speed internet with high latency. How do we make sure we serve them the proper data?
Throttle on low end devices
Lazy loading using CDN
Use a CDN
How do I iterate down the COLUMNS in a 2D array in JavaScript? (How is this different than iterating across the rows?)
In a 2D array made up of a box of 0s surrounded by 1s, how do I find the top left and bottom right coordinates? (LP)
For loop inside a for loop and look for the 0s? The first 0 is top left, the last 0 is bottom right
What are hooks?
Hooks are special functions in React that let you use features like state and lifecycle methods inside functional components.
Explain state management.
State management in React refers to how you handle dynamic data that changes over time in your application. Components manage their own local state using hooks like useState, but when multiple components need to share or update the same data, you either lift state up to a common parent or use the Context API or external libraries like Redux for global state. Efficient state management ensures components stay in sync and makes the app easier to maintain as it grows.
What is Non-null assertion in TypeScript? ([ ].find((unit) =>unit.id === item.id)!.done = !item.done)
It is a way to tell the compiler "this expression cannot be null or undefined here, so don't complain about the possibility of it being null or undefined." Sometimes the type checker is unable to make that determination itself.
The bang operator tells the compiler to temporarily relax the "not null" constraint that it might otherwise demand. It says to the compiler: "As the developer, I know better than you that this variable cannot be null right now".
from October 2024 Study Group session
What is React and how is it different from other frameworks (like Angular)?
React is a JS library, differs based on component structure. Angular is MVC, React is parent > child. React utilizes the Virtual DOM. React updates faster
What are the two different types of React components?
Functional:
Uses hooks, including for state (useState, useEffect, etc.)
Generally more succinct, as far as lines of code
Class-based:
Classic way of doing React
State is stored in single object/function
Uses lifecycle methods
What makes a functional React component stateless? (from previous question)
hooks store state information, as opposed to traditional state function.
Explain what the component lifecycle is.
Mounting: when the component gets loaded onto the page
Updating: the component changes what is shown on the page based on state, actions the user takes, etc.
Unmounting: the component is removed from the DOM. Usually happens on hard reload, new page/URL, etc.
What is re-rendering in React/what causes it?
When a component UI updates based on state changes.
What is Redux?
State management middleware
predictable state container for JavaScript applications
helps manage the application's state in a centralized way, making it easier to understand and debug.
Redux promotes a unidirectional data flow, making state management more predictable and easier to trace through the application's lifecycle. It's particularly useful in larger applications with complex state requirements.
What could you use in place of Redux?
Relay
Other middleware libraries
Context API - maybe could use it based onx complexity of app
Zustand
What are react hooks? And how did you use them in your last project?
React functions that are the replacement for more traditional lifecycle methods in class-based components.
Examples of hooks:
useState: establish state variables
useEffect: fetch data, manage side effects based on dependencies
useReducer: can be used with Redux or other middleware
useRef: can grab a piece of the DOM and manipulate it
useContext: access context values without needing to wrap components in a Context.Consumer
useCallback & useMemo: optimize performance by memoizing values and functions, preventing unnecessary recalculations on renders.
Can create custom hooks and use them throughout your app
What is the difference between `const` and `var` and `let`?
const: value cannot be changed or reassigned. Block scope.
let: value can be changed. Preferred over var. Block scope. Does not move to top of scope at execution (ie. does not “bubble up”).
var: value can be changed, not block scoped (ie. variable can have different values in different scopes). Moved to top of scope at execution (hoisting).
Temporal dead zone: In JavaScript, the Temporal Dead Zone (TDZ) is the area of a block where a variable declared with let or const exists but cannot be accessed. It starts at the beginning of the block and ends when the variable is declared and initialized.
How does the JavaScript event queue work?
What are prototypes and how does prototypal inheritance work?
What are closures and how are they related to data encapsulation? (Ash)
How do promises work? (Ash)
What is the difference between debounce and throttle?
Debouncing postpones the execution until after a period of inactivity or idle time. It’s helpful for delaying a function call for something like when waiting for a user to finish typing to execute.
Throttling limits the execution to a fixed number of times over an interval. [Medium Article]
What is the difference between callbacks and promises?
Callbacks call to another function, promises are run to resources outside the program (like http request) waiting for a value to come back
Callback hell? Can get super nested
Both can handle asynchronous tasks, but callbacks do it within nested functions while Promises are a cleaner way to await return value
Promises return Promise return type -> pending, fulfilled, rejected
Promises can be chained - automatically asynchronous
Callback has to be explicitly stated as asynch
From MDN Docs: Essentially, a promise is a returned object to which you attach callbacks, instead of passing callbacks into a function.
Implement Promise All.
Wait until you get all the calls back. Wait until it’s done
Example: construct an array of Promises and use Promise.all, if one fails, short circuits & whole thing is rejected
related: Promise.allSettled
Rejects immediately if any of the input Promises rejects
To reimplement assuming Promise and Promise.all don’t exist:
take in a list of function calls to make
run each of the functions / fetch requests in a loop or asynchronously depending on the question parameters (or use Promise if you still have that)
keep track of the return statuses
return the list of results once all have completed
Or return an error if some fail
Another version of the question: important that they can finish in any order → use threads
Example of using Promise.all:
const promises = wishlistItems.map((item) => {
const url = `http://localhost/api/wishlistItems/${item.id}`;
const deleteItemReponse = customFetch(url, options);
return deleteItemReponse;
});
await Promise.all(promises);
What happens when someone clicks a link in a browser?
Good initial questions for clarity: are we talking about links to external resources, links navigating within the webpage / single page app, etc
For external urls it’s like loading a page: Looks up IP address (DNS lookup). Browser initiates TCP connection with server. Once a connection is established, the browser sends an HTTP request to the resource. The server processes the request and sends back a response. The browser then renders the response data.
For "What happens when someone clicks a link in a browser?", this article (https://dev.to/katielee/what-really-happens-when-you-click-on-a-link-29o4) references the address portion and how it would identify the next steps. I'm guessing this question would assume that the path is absolute like we talked about though
If you’re fetching data on a page load, how would you do that in React?
useEffect with empty brackets [ ] as the second parameter executes the fetch on mount
const fetchData = async (topic) => {
setData(await getTopic(topic));
};
useEffect(() => {
fetchData(topic);
}, []);
Recommendation: put useEffect on page load as high as possible in the component hierarchy (the most parent). If you have multiple components all fetching on page load this can cause bugs if the returns of those async calls are affecting the same components. (e.g. 2 different pages that both check for login status)
Put the actual fetch call elsewhere, not directly in the useEffect
What is the return value of useEffect used for? follow up: How do you use setTimeout with useEffect?
Used for cleanup. Has same functionality as the legacy component unmount lifecycle method.
A common use case is to clean up timers, or to cancel / abort pending requests
useEffect(() => {
const timeoutId = setTimeout(() => {
setMessage('Message updated after 2 seconds');
}, 2000);
return () => {
clearTimeout(timeoutId); // Clear the timeout on unmount
};
}, []); // Empty dependency array ensures this runs only once on mount
When would you use React contexts?
Used to pass data in the component tree to avoid excessive prop drilling (passing props through deeply nested children).
Functional React + Contexts seem to have replaced Redux as a more lightweight way to utilize state from other parts of your application
useState is like component-based state, useContext is like Redux
Can still be used in class components through <Context.Consumer> wrapper component
Why are people moving away from Redux?
Not a question but have been asked for general familiarity with using fetch in javascript?
What is a ref?
How to implement a (anchor) tag in react jsx?
What is Context API, and when would you use it?
What is lazy loading in React, and how can you implement it?
How do you perform a state update that takes the previous state into account? A code question so not sure on phrasing, but getting at passing an updater function to setState()
What is memoization?
When to use useEffect vs useMemo?
When to use useContext versus Redux
When to use useMemo and useCallback
Definition of useMemo and useEffect?
How would you optimize a React application?
What is middleware and its function in React / how have you used it?
What kind of performance considerations do you keep in mind when writing React?
When to use ReactQuery versus Redux
Why do we update state with a brand new object, instead of updating the existing object? What would happen if we did update the state with the existing object?
What is local storage vs session storage and how do you access both?
from November 2023 Frontend Study Group
What is the Virtual DOM?
The Virtual DOM is a lightweight JavaScript representation of the Real DOM (Document Object Model). While the Real DOM is the actual structure used to display content in the User Interface, the Virtual DOM acts as an in-memory representation. This allows React to update only the necessary parts of the UI, improving performance and speeding up rendering.
What are props?
Props, short for properties, are used in React to pass data from a parent component to a child component. They enable dynamic customization and configuration of components. Props are immutable, meaning the child component cannot modify the prop values received from its parent.
What is the difference between a pure component and a component using shouldComponentUpdate?
A PureComponent in React automatically performs a shallow comparison of state and props to determine whether a re-render is necessary, eliminating the need for the shouldComponentUpdate() lifecycle method. In contrast, a regular component using shouldComponentUpdate requires you to manually decide when re-rendering should occur, offering more control but requiring more effort to manage.
What are controlled vs. uncontrolled components?
Controlled Component: The component’s state is controlled by React, typically via the parent component. When a user interacts with the component, React updates the state and re-renders the component.
Uncontrolled Component: The component manages its own internal state. You access the DOM directly via a ref to retrieve and update values.
Pros/Cons:Controlled Components: Easier to debug and predictable but can be complex to implement with deeply nested components.
Uncontrolled Components: Simpler to implement but harder to manage and test due to their internal state.
What are the pros and cons of Server-Side Rendering (SSR)?
Pros:
Improves performance by rendering the page on the server before sending it to the client (faster initial paint).
Enhances data privacy and security, though this must be implemented with intent.
Cons:
Higher latency due to server-side processing.
Read more.
How do you implement Server-Side Rendering (SSR) in React?
SSR in React can be implemented using tools like Webpack and Babel, along with frameworks such as Next.js, which simplifies the setup for server-side rendering.
Have you worked with state management?
Yes, I’ve used React Redux with both Thunks and Sagas for handling state. Additionally, React Context provides a simpler way to pass data through the component tree.
How do you improve React performance?
Use memoization with useMemo() and useCallback().
Optimize images and implement caching.
Utilize SSR for faster initial page load.
Next.js for built-in SSR features.
Tools like Lighthouse can audit page performance, particularly for time-to-paint metrics.
Learn more.
How do you encapsulate packages?
In a frontend development context, encapsulation refers to organizing related code, styles, and assets into cohesive, reusable modules or components. This can be achieved through:
Module Systems: Using CommonJS or ES6 modules to separate functionality into different files.
Package Managers: Managing dependencies with npm or Yarn.
Bundlers: Tools like Webpack or Parcel help bundle and optimize code for deployment.
Component Architecture: React components encapsulate functionality, styles, and markup.